How to understand the return values of scipy.interpolate.splrep
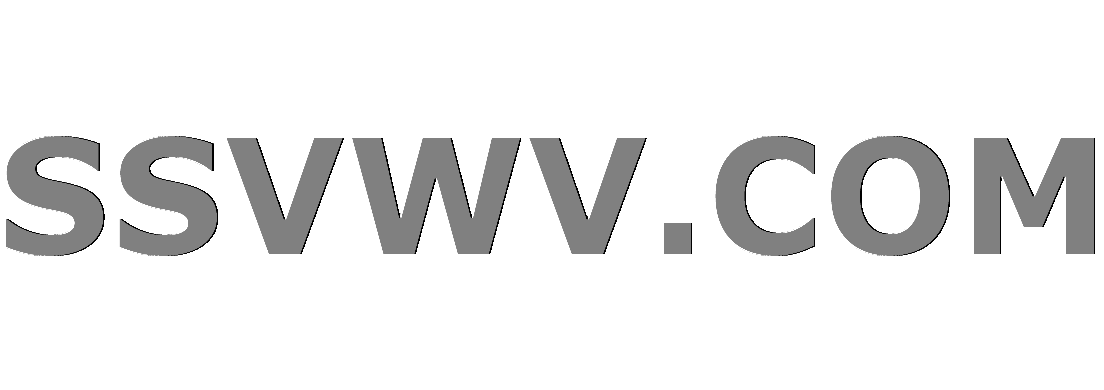
Multi tool use
$begingroup$
Background
Continuation of Spline interpolation - why cube with 2nd derivative
as following Cubic Spline Interpolation in youtube. The example in the youtube is below.
Implemented using scipy.interpolate.splrep and try to understand what the returns of the splrep function are.
Given the set of data points (x[i], y[i]) determine a smooth spline approximation of degree k on the interval xb <= x <= xe.
Returns
tck : tuple
A tuple
(t,c,k) containing the vector of knots, the B-spline coefficients, and the degree of the spline.
import numpy as np
from pylab import plt, mpl
plt.style.use('seaborn')
mpl.rcParams['font.family'] = 'serif'
%matplotlib inline
def create_plot(x, y, styles, labels, axlabels):
plt.figure(figsize=(10, 6))
for i in range(len(x)):
plt.plot(x[i], y[i], styles[i], label=labels[i])
plt.xlabel(axlabels[0])
plt.ylabel(axlabels[1])
plt.legend(loc=0)
x = np.array([3.0, 4.5, 7.0, 9.0])
y = np.array([2.5, 1.0, 2.5, 0.5])
create_plot([x], [y], ['b'], ['y'], ['x', 'y'])
import scipy.interpolate as spi
interpolation = spi.splrep(x, y, k=3)
IX = np.linspace(3, 9, 100)
IY = spi.splev(IX, interpolation)
create_plot(
[x, IX],
[y, IY],
['b', 'ro'],
['x', 'IY:interpolation'],
['x', 'y']
)
Questions
How to interpret and understand the return values and which resources to look into to understand?
A tuple (t,c,k) containing the vector of knots, the B-spline coefficients, and the degree of the spline.
The return value on Knots
interpolation[0]
array([3., 3., 3., 3., 9., 9., 9., 9.])
I thought the first tuple element would be the knots which would be the x, but not. What are these 3., 3. ... values?
The return values on B-spline co-efficient
interpolation[1]
array([ 2.5 , -2.21111111, 6.18888889, 0.5 , 0. , 0. , 0. , 0. ])
Please help or suggest where I should look into and what to understand about "B-spline coefficient" to be able to interpret these values?
The solution of the first interval is (0.186566, 1.6667, 0.24689), hence I thought these values would be in the 2nd element, but not. How the solution values would relate to the return values?
scipy interpolation
$endgroup$
add a comment |
$begingroup$
Background
Continuation of Spline interpolation - why cube with 2nd derivative
as following Cubic Spline Interpolation in youtube. The example in the youtube is below.
Implemented using scipy.interpolate.splrep and try to understand what the returns of the splrep function are.
Given the set of data points (x[i], y[i]) determine a smooth spline approximation of degree k on the interval xb <= x <= xe.
Returns
tck : tuple
A tuple
(t,c,k) containing the vector of knots, the B-spline coefficients, and the degree of the spline.
import numpy as np
from pylab import plt, mpl
plt.style.use('seaborn')
mpl.rcParams['font.family'] = 'serif'
%matplotlib inline
def create_plot(x, y, styles, labels, axlabels):
plt.figure(figsize=(10, 6))
for i in range(len(x)):
plt.plot(x[i], y[i], styles[i], label=labels[i])
plt.xlabel(axlabels[0])
plt.ylabel(axlabels[1])
plt.legend(loc=0)
x = np.array([3.0, 4.5, 7.0, 9.0])
y = np.array([2.5, 1.0, 2.5, 0.5])
create_plot([x], [y], ['b'], ['y'], ['x', 'y'])
import scipy.interpolate as spi
interpolation = spi.splrep(x, y, k=3)
IX = np.linspace(3, 9, 100)
IY = spi.splev(IX, interpolation)
create_plot(
[x, IX],
[y, IY],
['b', 'ro'],
['x', 'IY:interpolation'],
['x', 'y']
)
Questions
How to interpret and understand the return values and which resources to look into to understand?
A tuple (t,c,k) containing the vector of knots, the B-spline coefficients, and the degree of the spline.
The return value on Knots
interpolation[0]
array([3., 3., 3., 3., 9., 9., 9., 9.])
I thought the first tuple element would be the knots which would be the x, but not. What are these 3., 3. ... values?
The return values on B-spline co-efficient
interpolation[1]
array([ 2.5 , -2.21111111, 6.18888889, 0.5 , 0. , 0. , 0. , 0. ])
Please help or suggest where I should look into and what to understand about "B-spline coefficient" to be able to interpret these values?
The solution of the first interval is (0.186566, 1.6667, 0.24689), hence I thought these values would be in the 2nd element, but not. How the solution values would relate to the return values?
scipy interpolation
$endgroup$
add a comment |
$begingroup$
Background
Continuation of Spline interpolation - why cube with 2nd derivative
as following Cubic Spline Interpolation in youtube. The example in the youtube is below.
Implemented using scipy.interpolate.splrep and try to understand what the returns of the splrep function are.
Given the set of data points (x[i], y[i]) determine a smooth spline approximation of degree k on the interval xb <= x <= xe.
Returns
tck : tuple
A tuple
(t,c,k) containing the vector of knots, the B-spline coefficients, and the degree of the spline.
import numpy as np
from pylab import plt, mpl
plt.style.use('seaborn')
mpl.rcParams['font.family'] = 'serif'
%matplotlib inline
def create_plot(x, y, styles, labels, axlabels):
plt.figure(figsize=(10, 6))
for i in range(len(x)):
plt.plot(x[i], y[i], styles[i], label=labels[i])
plt.xlabel(axlabels[0])
plt.ylabel(axlabels[1])
plt.legend(loc=0)
x = np.array([3.0, 4.5, 7.0, 9.0])
y = np.array([2.5, 1.0, 2.5, 0.5])
create_plot([x], [y], ['b'], ['y'], ['x', 'y'])
import scipy.interpolate as spi
interpolation = spi.splrep(x, y, k=3)
IX = np.linspace(3, 9, 100)
IY = spi.splev(IX, interpolation)
create_plot(
[x, IX],
[y, IY],
['b', 'ro'],
['x', 'IY:interpolation'],
['x', 'y']
)
Questions
How to interpret and understand the return values and which resources to look into to understand?
A tuple (t,c,k) containing the vector of knots, the B-spline coefficients, and the degree of the spline.
The return value on Knots
interpolation[0]
array([3., 3., 3., 3., 9., 9., 9., 9.])
I thought the first tuple element would be the knots which would be the x, but not. What are these 3., 3. ... values?
The return values on B-spline co-efficient
interpolation[1]
array([ 2.5 , -2.21111111, 6.18888889, 0.5 , 0. , 0. , 0. , 0. ])
Please help or suggest where I should look into and what to understand about "B-spline coefficient" to be able to interpret these values?
The solution of the first interval is (0.186566, 1.6667, 0.24689), hence I thought these values would be in the 2nd element, but not. How the solution values would relate to the return values?
scipy interpolation
$endgroup$
Background
Continuation of Spline interpolation - why cube with 2nd derivative
as following Cubic Spline Interpolation in youtube. The example in the youtube is below.
Implemented using scipy.interpolate.splrep and try to understand what the returns of the splrep function are.
Given the set of data points (x[i], y[i]) determine a smooth spline approximation of degree k on the interval xb <= x <= xe.
Returns
tck : tuple
A tuple
(t,c,k) containing the vector of knots, the B-spline coefficients, and the degree of the spline.
import numpy as np
from pylab import plt, mpl
plt.style.use('seaborn')
mpl.rcParams['font.family'] = 'serif'
%matplotlib inline
def create_plot(x, y, styles, labels, axlabels):
plt.figure(figsize=(10, 6))
for i in range(len(x)):
plt.plot(x[i], y[i], styles[i], label=labels[i])
plt.xlabel(axlabels[0])
plt.ylabel(axlabels[1])
plt.legend(loc=0)
x = np.array([3.0, 4.5, 7.0, 9.0])
y = np.array([2.5, 1.0, 2.5, 0.5])
create_plot([x], [y], ['b'], ['y'], ['x', 'y'])
import scipy.interpolate as spi
interpolation = spi.splrep(x, y, k=3)
IX = np.linspace(3, 9, 100)
IY = spi.splev(IX, interpolation)
create_plot(
[x, IX],
[y, IY],
['b', 'ro'],
['x', 'IY:interpolation'],
['x', 'y']
)
Questions
How to interpret and understand the return values and which resources to look into to understand?
A tuple (t,c,k) containing the vector of knots, the B-spline coefficients, and the degree of the spline.
The return value on Knots
interpolation[0]
array([3., 3., 3., 3., 9., 9., 9., 9.])
I thought the first tuple element would be the knots which would be the x, but not. What are these 3., 3. ... values?
The return values on B-spline co-efficient
interpolation[1]
array([ 2.5 , -2.21111111, 6.18888889, 0.5 , 0. , 0. , 0. , 0. ])
Please help or suggest where I should look into and what to understand about "B-spline coefficient" to be able to interpret these values?
The solution of the first interval is (0.186566, 1.6667, 0.24689), hence I thought these values would be in the 2nd element, but not. How the solution values would relate to the return values?
scipy interpolation
scipy interpolation
asked 20 hours ago
monmon
1073
1073
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
$begingroup$
This documentation may work:
BSpline
Univariate spline in the B-spline basis.
$S(x) = sum_{j=0}^{n-1} c_jB_{j,k;t}(x)$
where $B_{j,k;t}$ are B-spline basis functions of degree k and knots t.
New contributor
Juan Esteban de la Calle is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "557"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fdatascience.stackexchange.com%2fquestions%2f49247%2fhow-to-understand-the-return-values-of-scipy-interpolate-splrep%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
This documentation may work:
BSpline
Univariate spline in the B-spline basis.
$S(x) = sum_{j=0}^{n-1} c_jB_{j,k;t}(x)$
where $B_{j,k;t}$ are B-spline basis functions of degree k and knots t.
New contributor
Juan Esteban de la Calle is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
This documentation may work:
BSpline
Univariate spline in the B-spline basis.
$S(x) = sum_{j=0}^{n-1} c_jB_{j,k;t}(x)$
where $B_{j,k;t}$ are B-spline basis functions of degree k and knots t.
New contributor
Juan Esteban de la Calle is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
This documentation may work:
BSpline
Univariate spline in the B-spline basis.
$S(x) = sum_{j=0}^{n-1} c_jB_{j,k;t}(x)$
where $B_{j,k;t}$ are B-spline basis functions of degree k and knots t.
New contributor
Juan Esteban de la Calle is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
This documentation may work:
BSpline
Univariate spline in the B-spline basis.
$S(x) = sum_{j=0}^{n-1} c_jB_{j,k;t}(x)$
where $B_{j,k;t}$ are B-spline basis functions of degree k and knots t.
New contributor
Juan Esteban de la Calle is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Juan Esteban de la Calle is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 19 hours ago
Juan Esteban de la CalleJuan Esteban de la Calle
938
938
New contributor
Juan Esteban de la Calle is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Juan Esteban de la Calle is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Juan Esteban de la Calle is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
Thanks for contributing an answer to Data Science Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fdatascience.stackexchange.com%2fquestions%2f49247%2fhow-to-understand-the-return-values-of-scipy-interpolate-splrep%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
rR,Ej77G8I I,ATH,FdRI3yqP